If you've followed my tutorials so far, you have created an application which shows a message box when you click a button that was placed on the form.
This tutorial will teach you how to work with variables. At a basic level, a variable is a storage space in your computer's memory. You can use variables to store numbers, characters (single letters), strings of characters (just like the one that was used for MessageBox in the previous tutorial), and so on.
It is best to define specific variables for specific uses. This means that you should create numerical variables to store numbers, and string variables to store strings. C# will also allow you to create a specific variable type, which helps you store all sorts of data; we'll discuss it as well.
It's time to start working! Start a new project, add a button to the form, and then double click it to show its code.
Here's what you should get.
Examining C# variables
Copyright
Matthew S. Alford
All Rights reserved.
- Custom small business software
- Affordable prices
Add the two lines of code below between the curly brackets:
string MyName = "Matt";
MessageBox.Show(MyName);
The first line of code defines a variable named "MyName". You can choose your own variable name, of course. The variable will be a "string", a memory space that is specialized in storing a set of characters. I have assigned that string variable my own name (Matt). Don't forget that each string must be surrounded by quotes, and that the line should end with a semicolon.
The second line of code will display a message box that shows the MyName string. This time we didn't write the actual name inside the paranthesis (Matt) because it was already stored inside the string variable. This way, if we decide to change the name later, we can replace it inside the MyName string definition, and the message box will update it accordingly.
Run the program, click the button and you should see a message box that displays my name.
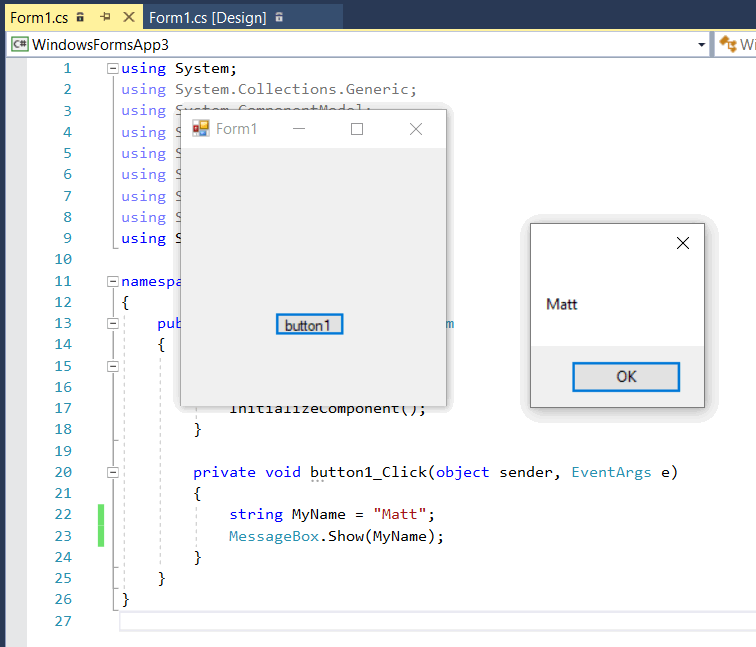
Go ahead, replace the name in the string definition with yours; I know you want to do that :)
The second variable type that we are going to discuss is the integer, a variable that can only store integer numbers. This means that if you'd do a 7:2 math operation and store the result in an integer, the answer to that division would be 3! Yes, I know that 7:2=3.5, but the integer variable can't store decimals.
Here's how you can define an integer:
int MyGrade = 9;
We begin our line of code with "int", followed by the desired variable name. The rest of the line assigns the MyGrade variable an initial value of 9, and everything ends with a semicolon.
You could try to display MyGrade using a MessageBox, but it wouldn't work, because MessageBox is used to display text (string-based) messages, and not variables. Don't lose hope, though, because there's an easy method of converting numbers (our int) into strings, which can then be used by MessageBox - ToString().
So, add these two lines of code inside the curly brackets, replacing the ones from the previous example.
int MyGrade = 9;
MessageBox.Show(MyGrade.ToString());
Run the application, click the button and you will see the value of MyGrade.
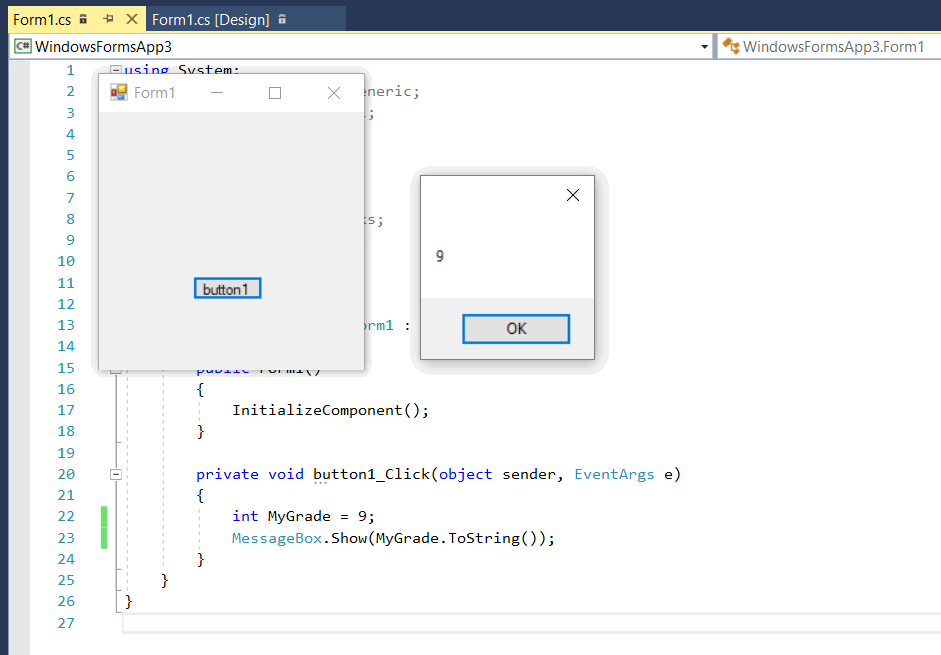
Feel free to play with various MyGrade values; the displayed value will change any time you edit it.
I have told you that C# also supports a variable that can store different data types. It's time to meet the "object" variable.
Here's how it works.
object anything = 9;
This is a typical "object" variable definition. It declares an object variable named "anything", and then it assigns it a value of 9.
Nothing unusual so far, right? Hold on just a little bit!
object anything = "Matt";
Did you see what happened above? We've got the same object definition, using the same name, but this time we assign it a string value. Our MessageBox instruction will be able to display both values (one at a time, of course) without erroring out.
Here's how my source code file looks like now.
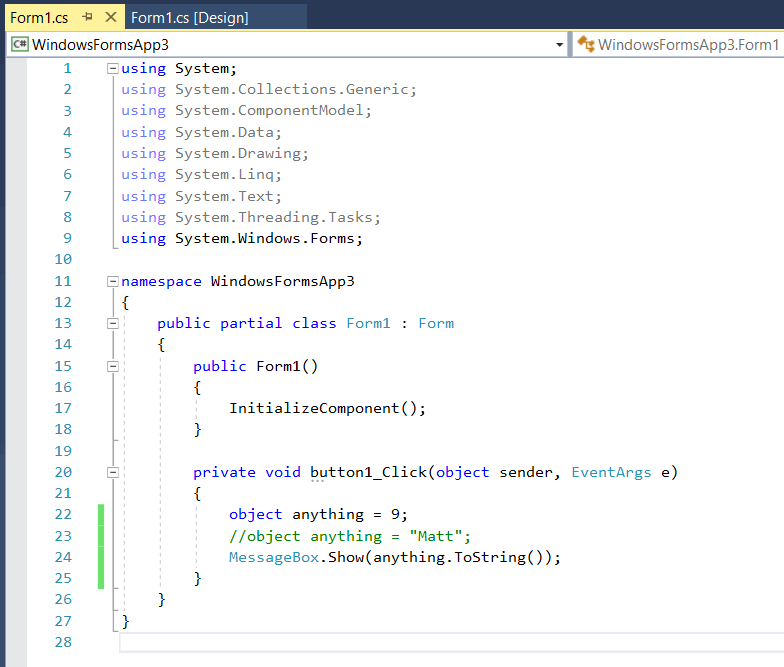
As you can see, I am commenting one of the "object" definitions. Run the application, examine its output, and then stop it. Comment the first "object" definition, uncommenting the second one, and then run the program again. You will see that the app will display "9" and "Matt" correctly, even though the variable uses two different data types.
This wraps up our C# variables tutorial. Next time we will look into powerful methods that allow us to change the aspect and properties of our forms by writing a few lines of code. This way, you will be able to customize the user interface at runtime, move the form around using code, and much more.